Let us write some C++ Programs
Operation | Symbol used in C++ |
Addition | + |
Subtraction | - |
Multiplication | * |
Division | / |
Remainder operation | % |
Things to be done to write the program
(i) We need three variables to store the three numbers, one variable to store the result
(ii) Write codes to make the computer to accept 3 numbers.
(iii) Write codes to add the numbers.
(iv) Write code to display the result
For the first task we will declare 4 variables (int variable), for the second task we will use "cin" command, for the third task we will use addition (+) operators.
For the last task we will use "cout". Since we will use "cin" and "cout" we have to include "iostream.h". Let us write the program-
Program-1
#include <iostream.h> void main(){ int a, b, c, d; cin>>a; cin>>b; cin>>c; d=a+b+c; cout<<d; }Now if this program is run we will get an empty screen with a blinking cursor as shown below. Actually computer is waiting to receive the three numbers we are suppose to give because of those three "cin" in the program. This program is not at all user friendly, we have to guide the user properly how to operate the program. Therefore we will modify it next so that it becomes a user friendly program.
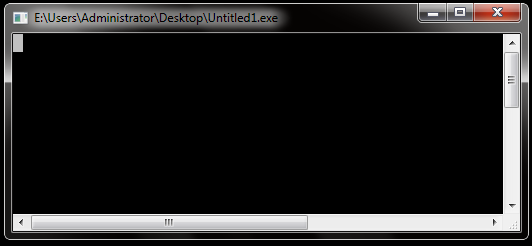
#include <iostream.h> void main(){ int a, b, c, d; cout<<"Enter the first No:"; cin>>a; cout<<"Enter the second No:"; cin>>b; cout<<"Enter the third No:"; cin>>c; d=a+b+c; cout<<"Sum="<<d; }Now compile the program and run it you will the output in Dev C++ as below -
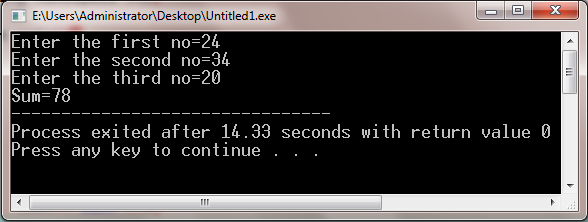
This time it will not display empty screen, but it will display the message "Enter the first no=" as we have given in the program. So enter the number and press the enter key, it will asks for second number and so on. Actually when we type first number and press enter key the variable "a" gets its value means a=24 as per the figure given and similarly all the variable gets its value successively; finally it calculates the sum and display by the "cout" command. The word "sum" before the result may be any word to convey the result, it may be "Result", "Answer" etc. There is no harm if nothing is given, but your program remain less user friendly.
Program- 1 in C
#include <stdio.h> void main(){ int a, b, c, d; printf("Enter the first No:"); scanf("%d",&a); printf("Enter the second No:"); scanf("%d",&b); printf("Enter the third No:"); scanf("%d",&c); d=a+b+c; printf("Sum=%d",d); }Point to remmember while programming in C : While using scanf statement do not forget to prefix the variable name with &. Use proper conversion specifiers like "%d" - for int; "%f" - for float; "%c" - for char; "%s" - for string (character array) type variable.