Parameter Passing Technique in C++
As you have seen in the previous praogram we have passed two parameters to the function "sum()"- there are two ways to pass parameters to a function.
1. Parameter Passing by Value.
2. Parameter Passing by Reference
Parameter Passing by Value: In this technique a copy of the parameters (not the original) are passed to the function, so if there is any change in the parameters it would not be visible from the calling function
Parameter Passing by Reference: In this technique the address of the parameters are passed to the function, so if there is any change in the parameters it would be visible from the calling function
Let us try to undersatnd the techniques by example-
Program-63
#include <iostream.h> void Func1(int x, int y){ x=x+10; y=y+20; } void Func2(int &x, int &y){ x=x+10; y=y+20; } void main(){ int a, b; cout<<"Enter the No-1:"; cin>>a; cout<<"Enter the no-2:"; cin>>b; Func1(a,b); cout<<"Output after Func1 call\n"; cout<<"a="<<a<<"\n"; cout<<"b="<<b<<"\n\n"; Func2(a,b); cout<<"Output after Func2 call\n"; cout<<"a="<<a<<"\n"; cout<<"b="<<b<<"\n"; }See the output as below
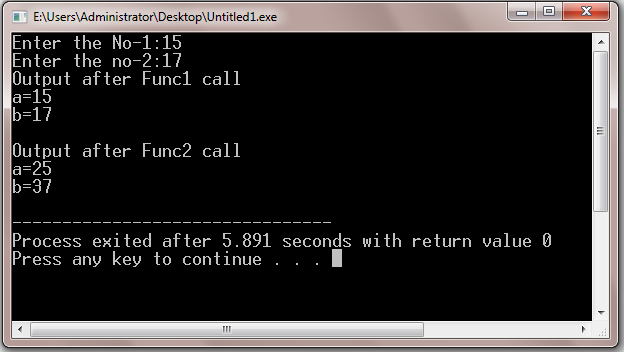
When the Func1() function is called the parameters "a", "b" are passed by value, means the copies of the variable "a" and "b" ( you can imagin "x" and "y" are different from "a" and "b" ) are passed to the function, hence the change done on the parameters as "x=x+10" and "y=y+20" in the function has not changed the values of "a" and "b" in the "main()" function, change is done on their copies (a' & b'). On the otherhand see function Func2() where the parameters are declared as reference parameters by preceding them by & sign. So while calling the function Func2() the address of the variable "a" and "b" are passed to the function (you can think "x" and "y" of the function is same variable as "a" and "b" of the "main()" function ) hence the change done on the parameters as "x=x+10" and "y=y+20" in the function has changed the values of "a" and "b" in the "main()" function. See the following diagram-
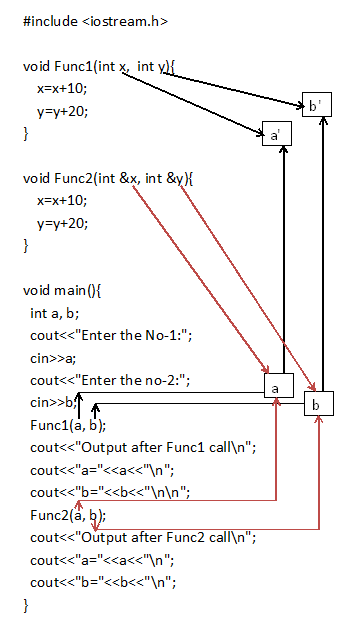
In the above diagram a' and b' are the copies of variable "a" and "b".
Local & Global Variables in C++
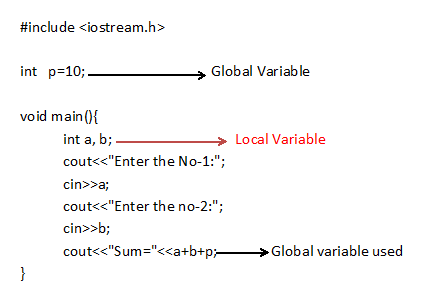
When global variable and local variable have the same name then the global variable is specified by preceeding the variable name by '::' (scope resolution operator see the diagram below-
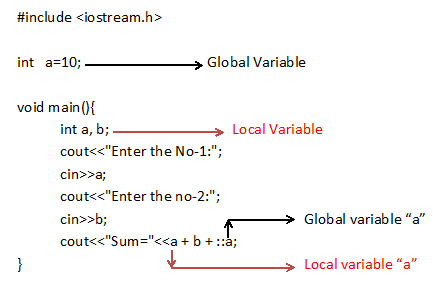
Default Parameter in C++
int func1(int a, int b=0, int c=0) here "b" and "c" are default parameters. While calling the function as func1(5), values for parameters "b" and "c" are not supplied so the default value would be used. But if we call the function as func1(2,4,6) then the supplied values would be used.
func2(int a=0, int b) is not allowed as default parameter "a" is not defined as the rightmost parameter. The correct declaration is func2(int b, int a=0).
Points to be remembered
- Function can return one value to the calling function
- Parameter can be passed in two ways - by value and by reference
- If we need that the function to return more than one value to the calling function then we have to use reference parameters
- Default parameters must be declared at the right side of the parameter list