Function in C++
By now we have used many functions in the programs, but those functions are compiler defined and part of C++ compiler, e.g sqrt(), strlen(), pow() etc. In this chapter we will study user defined functions means we will define our functions.
Function:A function is a sub-program that does a part of the whole task. The main advantage of a function is its re-usability- means when a function is defined it can be called (used) as many times as we want. Do not confuse with function and loop, loop repeats a block of statement in one place of the program, but function is called from different locations of a big programs. In simple words a function is nothing but a group of statement, these get executed when the function is called.
Part of a function
- Return type - a function has to return a value - int, float, char, double etc. If nothing required then "void" may be used
- Function name - follows the rules of variable naming
- Parameter/ arguments list with type - parameters are the values send to a function, ecah parameter must be declare with its type individually separated by comma.
- Function body - A group of processing statements
return_type function_name ( data_type para1, data_type para2 ...){ Statement - 1; Statement - 2; Statement - 3; . . . return ( value/ variable); }
Now the place in the program where the function is written - there are two places (1) write before the "main" function; (2) write after the "main" fumction, if written after main function then the function prototype to be declared in the "main" function. Function prototype is an indication that there is a function would be called from this function ( where the prototype is defining) is defined below. We would be following first method ie functions would be defined betwen <header files> and "main()" function.
Let us start with an exampl
Q62.Write a program to accept two numbers then find sum and average using function.
Program-62
#include <iostream.h>
int sum(int x, int y){
int z;
z=x+y;
return z;
}
void main(){
int a, b, c, av;
cout<<"Enter the No-1:";
cin>>a;
cout<<"Enter the no-2:";
cin>>b;
c=sum(a,b);
cout<<"The sum="<<c;
av=sum(a,b)/2.0;
cout<<"Average ="<<av;
}
In the above program see that function sum() is defined between <header file> and "main()" function. The function has two
parameters x & y both type int, the function returns the sum. Parameters that appear in function definition are called formal parameters and the parameters that appear in the function call ( in the main see sum(a,b)) are called actual parameters. See the re-usability of sum function while finding average.
Let us see how the program works-
See the figure below-
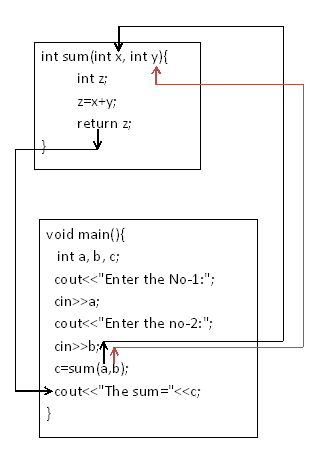
Remember that the variables(arguments/ parameters) in the function definition are alltogether different from the variables in the "main" functions, therefore if we take the same variable name (a, b ) in both places there is no harm.