Programing with if-else in C++
Q10.Write a C++ program to accept a number then display if it +ve, -ve or zero.
Q11.Write a C++ program to accept a number then check if it is odd or even.
Q12.Write a C++ program to accept two numbers then check if the first one is divisible by second one.
Q13.Write a C++ program to accept percentage of marks then display grade base on the following criteria.
% age of marks | grade |
>90 | A1 |
>80 but <=90 | A2 |
>70 but <=80 | B1 |
>60 but <=70 | B2 |
>50 but <=60 | C1 |
>40 but <=50 | C2 |
>=33 but <=40 | D |
otherwise | E |
Q15.Write a C++ program to accept the coefficients of a quadratic equation then find the roots of the equation.
Program-9
#include <iostream.h> void main(){ int age; cout<<"Enter the Age:"; cin>>age; if(age>=18){ cout<<"Congratulations You can Vote !!"; } else{ cout<<"Sorry You can not Vote"; } }
Program-10
#include <iostream.h> void main(){ int a; cout<<"Enter the number:"; cin>>a; if(a>0){ cout<<"The number is Positive"; } else if(a<0){ cout<<"The number is Negative"; } else{ cout<<"The number is Zero"; } }
Program-11
#include <iostream.h> void main(){ int a; cout<<"Enter the number:"; cin>>a; if(a%2==0){ cout<<"The number is Even"; } else{ cout<<"The number is Odd"; } }Note: In program-10, the given number is divided by 2 and its remainder is checked, if it is zero then the given number is "Even" otherwise it is "Odd". See "a%2==0" in the program.
#include <iostream.h> void main(){ int a, b; cout<<"Enter the first number:"; cin>>a; cout<<"Enter the second number:"; cin>>b; if(a%b==0){ cout<< a<<" is divisible by "<<b; } else{ cout<< a<<" is not divisible by "<<b; } }Output of Program-12
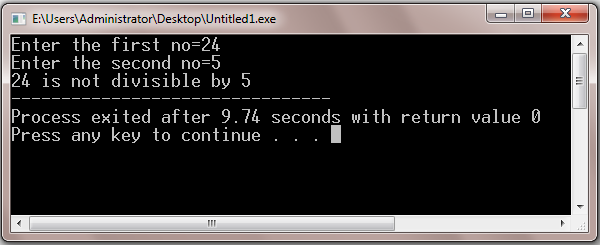
Program-13
#include <iostream.h> void main(){ int p; cout<<"Enter %age of marks:"; cin>>p; if(p>90){ cout<<"Grade is A1"; } else if(p>80){ cout<<"Grade is A2"; } else if(p>70){ cout<<"Grade is B1"; } else if(p>60){ cout<<"Grade is B2"; } else if(p>50){ cout<<"Grade is C1"; } else if(p>40){ cout<<"Grade is C2"; } else if(p>=33){ cout<<"Grade is D"; } else{ cout<<"Grade is E"; } }Note: In program-13, see that we have not checked the range of percentage like 80 to 90, but we have checked for "p>80". Let us take the percentage is 91 then one may think that 91 is greater than 90 as well as greater than all other goven conditions given in the program therefore it will display all the grades. Actually the first "if"block will be excuting and there is no chance that other blocks will also be executing as in an "if-else" construct only one block of statement executes at a time.
Program-14
#include <iostream.h> void main(){ float price, tot, dis, net; int qty; cout<<"Enter unit price:"; cin>>price; cout<<"Enter quantity purchased:"; cin>>qty; tot=price*qty; if(tot>=5000){ dis=tot*10/100; } else if(tot>=4000){ dis=tot*5/100; } else if(tot>=3000){ dis=tot*3/100; } else{ dis=0; } net=tot-dis; cout<<"Total Amount="<<tot<<"\n"; cout<<"Discount Amount="<<dis<<"\n"; cout<<"Net Amount payable="<<net; }Program-15
#include <iostream.h> #include <math.h> void main(){ float a, b, c, d, r1, r2; cout<<"Enter coefficient of square term:"; cin>>a; cout<<"Enter coefficient of x"; cin>>b; cout<<"Enter the constant-c"; cin>>c; d=b*b-4*a*c; if(d<0){ cout<<"Roots are Imaginary" } else{ r1=(-b+sqrt(d))/(2*a); r2=(-b-sqrt(d))/(2*a); cout<<"Root-1="<<r1<<"\n"; cout<<"Root-2="<<r2; } }Output of Program-15
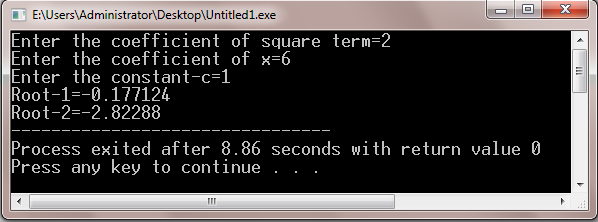