Flow of control in C++
In this chapter we will study few C++ commands on flow of control. These commands are fundamental commands and these are always used in programs. Therefore study this chapter carefully.
if-else - This a decision making command or statement. This statement divides a liner program into two paths based on a given condition. Before we use the statement let us study what a condition is.
let us take two integers "a" and "b".
Now what is the value of a+b ? Since "a" and "b" are integer then a+b must be integer, the set of integer is an infinite set so a+b may have infinite values.
Now if I ask you what is a>b? See whatever may the value of "a" and "b" , a>b would be always either "true" or "false" only, it is not infinite. This (a>b or a<=b) kind of expression is called relational expression or a condition, the first kind of expresion (a+b) is called arithmetic expression.
Operation | Operator in C++ |
Equal to | == |
Less than | < |
Grater than | > |
Less than equal to | <= |
Greater than equal to | >= |
Not equal to | != |
Note: For checking equality condition we have to use two equal to sign without any gap between them, e.g "a==b" checks is value of "a" is equal to the value of "b". Here let me tell you about another operator "=" which is called assignment operator. When we write "a=10" then variable "a" gets the value 10 or more technically 10 is stored in the memory locaton marked as "a".
if( condition ){ statement 1; statement 2; . . } else{ statement 10; statement 11; . . }
Multiple if-else statement syntax
if( condition 1 ){ statement 1; statement 2; . . } else if( condition 2 ){ statement 10; statement 11; . . } else if( condition 3 ){ statement 20; statement 21; . . } else{ statement 30; statement 31; . . }Note:
- Here the condition is a relational espression ( like a>10 ) must be written within parenthesis "( )" as given in the syntax and no semicolon after the closing parenthesis.
- If the relational expression evaluates to "true" then the statement block { statement 1 , statement 2 . . . } would be executing and it will skip statement block { statement 10 , statement 11 . . . }.
- If the relational expression evaluates to "false" then the statement block { statement 10 , statement 11 . . . } would be executing and it will skip statement block { statement 1 , statement 2 . . . }.
- The main point is that at anytime only one block of statement would be executing.
- If there is only one statement to be written within "if" statement then we can avoid the curly bracket pairs, same thing applies in "else" block. Therefore if you write more than one statements for "true" condition and you forget to enclosed it within curly bracket pairs then only one statement would be considered for it. That is why it is advisable (at least for the beginners) to use the bracket pairs.
Program-8
#include <iostream.h> void main(){ int a, b; cout<<"Enter the first no:"; cin>>a; cout<<"Enter the second no:"; cin>>b; if(a>b){ cout<<"Greater No is"<<a; } else{ cout<<"Greater No is"<<a; } }Output of Program-8
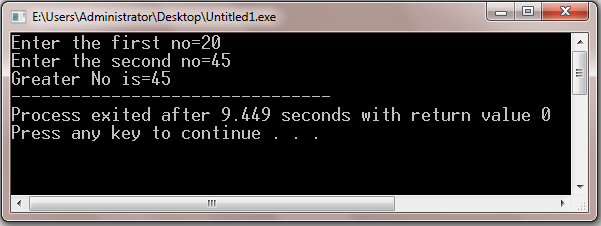