2 Dimenssional Array in C++
In a two dimenssional array there are rwos and colums. It is declared in C++ as -
int a[5][4]; in this array there are 5 rows and 4 colums. As usual row index ranges from 0 to 4 and column index ranges from 0 to 3. This declaration will make a table in the memory(RAM) as shown below-
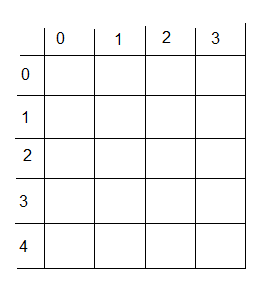
The numbers at the top specifies column index number and numbers at the left side specifies row index number. e.g a[2][1] specifies elsement at third row (index number 2) and 2nd column (index number 1). The total number of elements in the array "a" is 20 (5x4).
Now let us write few programs using array-
Q57.Write a program to accept a nXn matrix then find the sum of the diagonal elements.
Q58.Write a program to accept a mXm matrix the tramspose it.
Q59.Write a program to accept a 5x5 matrix then find the sum of all the rows.
Q60.Write a program to accept a 5x5 matrix then find the sum of all the columns.
Q60.Write a program to find sum of two 4x4 matrix.
Program-57
#include <iostream.h>
void main(){
int ar[10][10], i, j, r, sum=0, k, cm;
cout<<"Enter number of rows or cols:";
cin>>r;
for(i=0; i<= r-1; i++){
for(j=0; j<=r-1; j++){
cout<<"Enter the element at ("<<i<<","<<j<<")=";
cin>>ar[i][j];
}
}
k=r-1;
for(i=0; i<=r-1; i++){
sum=sum+ar[i][i]+ar[i][k];
k--;
}
if(r%2==1){
cm=(r-1)/2;
sum=sum-ar[cm][cm];
}
cout<<"Sum="<<sum;
}
In this program the array is define as "ar[10][10]" as we have to accept the size of the matrix from the user. Here user has the enter the size of the square matrix, so any one of row or column number is accepted from the user. Then the elements are accepted from the user. Assuming that user entered a 5X5 matrix then the diagonal elements are (from left to right) at (0,0), (1,1), (2,2), (3,3), (4,4) and the elements of the other diagonal (right to left) are (0,4), (1,3), (2,2), (3,1), (4,0). See that the element at (2,2) are common in both the diagonals. When the order of the matrix is odd then there would be a common element. Now see in the program we have taken a variable k which is assigned to the last colum nuber of the matrix (for 5x5 matrix k=4). See in the loop where the sum operation is done the term ar[i][i] generating the terms of first diagonal elements and the term a[i][k] generating the terms of the second diagonal. The last "if" statement is checking if the order of the matrix is odd, if yes it is subtracting the common element from the sum.
#include <iostream.h> void main(){ int ar[10][10], i, j, r;; cout<<"Enter number of rows or cols:"; cin>>r; for(i=0; i<= r-1; i++){ for(j=0; j<=r-1; j++){ cout<<"Enter the element at ("<<i<<","<<j<<")="; cin>>ar[i][j]; } } cout<<"The original matrix is as under\n" for(i=0; i<= r-1; i++){ for(j=0; j<=r-1; j++){ cout<<ar[i][j]<<" "; } cout<<"\n"; } for(i=0; i<= r-1; i++){ //transpose for(j=0; j<=i; j++){ q=ar[i][j]; ar[i][j]=ar[j][i]; ar[j][i]=q; } } cout<<"The transposed matrix is as under\n" for(i=0; i<= r-1; i++){ for(j=0; j<=r-1; j++){ cout<<ar[i][j]<<" "; } cout<<"\n"; } }First of all try to understand the transpose operation of a matrix- in this operation rows are converted in columns and vice-versa. Again note that when we exchange values between two variable then we need a temporary variable, e.g assume a=4, b=8, now we exchange the values as- c=a, a=b, and b=c see the figure below. In this program also the same technique is used in the segment mark as "transpose", the temporary variable is "q". Note that the second for loop under "transpose" segment goes from 0 to i, see the element at (i, j) and element at (j, i) are interchanged
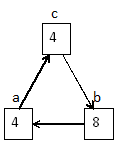
#include <iostream.h> void main(){ int ar[10][10], i, j, r, c, sum; cout<<"Enter number of rows:"; cin>>r; cout<<"Enter number of cols:"; cin>>c; for(i=0; i<= r-1; i++){ for(j=0; j<=c-1; j++){ cout<<"Enter the element at ("<<i<<","<<j<<")="; cin>>ar[i][j]; } } for(i=0; i<= r-1; i++){ sum=0; for(j=0; j<=c-1; j++){ sum=sum + ar[i][j]; } cout<<"Sum of the elements of row -"<<i<<":"<<sum; cout<<"\n"; } }In this program number of rows and columns are accepted from the user, then the matrix is generated by accepting the elements from the user. The variable sum is initialized within the loop (second set of loops) as we have found sum of each row individually.
Program-60
Study the program-59 and do it yourself.Program-61
#include <iostream.h> #include <conio.h> void main(){ int A[4][4], B[4][4], C[4][4],i, j; for(i=0; i<= 3; i++){ for(j=0; j<=3; j++){ cout<<"Matrix- A the element at ("<<i<<","<<j<<")="; cin>>A[i][j]; } } for(i=0; i<= 3; i++){ for(j=0; j<=3; j++){ cout<<"Matrix- B the element at ("<<i<<","<<j<<")="; cin>>B[i][j]; } } clrscr(); cout<<"The Matrix A \n";//Displaying A for(i=0; i<= 3; i++){ for(j=0; j<=3; j++){ cout<<A[i][j]<<" "; } cout<<"\n"; } cout<<"The Matrix B \n"; //Displaying B for(i=0; i<= 3; i++){ for(j=0; j<=3; j++){ cout<<B[i][j]<<" "; } cout<<"\n"; } for(i=0; i<= 3; i++){ //Fiding sum for(j=0; j<=3; j++){ C[i][j]=A[i][j] + B[i][j]; } } cout<<"The Matrix B \n"; //Displaying C for(i=0; i<= 3; i++){ for(j=0; j<=3; j++){ cout<<C[i][j]<<" "; } cout<<"\n"; } }This program is reading two matrices A & B and adding them to make Matrix C
Write the following program yourself
Q. Wrire a program to multiply a matrix by a scalar .
Q. Write a program subtract a matrix from another and store the result in a third matrix ( like addition of matrix)