Array in C++
Introduction:Let us start with an example- suppose you have to write a program to accept 10 integers and sort it in ascending order. Now in the program if you take 10 individual variables as a, b, c etc. Then can you think of a logic to sort the numbers? It would be a very trublesome job. The problem is that the variables are not related by any relation, as they are created in the memory (RAM) in different locations controlled by other programs. You can not use any loop as you can not generalized the process. In this situation we have to use Array which can store the numbers and all the numbers are stored in successive memory locations. If we know any one of the numbers then we can find the others, so we can use loop.
Array:Technically an array is a collection of similar data which share same name. Each data item is identified by its location (index number) in the array.
Syntax of declaration : datatype name_of_array[ no_of_elements];
e.g int a[10], it will create an array of 10 integers whose single name is "a". Actually in this array "a" we have 10 integer variables they are - a[0], a[1], a[2]...a[9]. Therefore we can accept numbers in each of them using "cin" statemet, we can assign any number to them e.g a[0]=10. Same way we can declare array of type float, double, char.
See the figure below-

When we declare an array as int a[10] and compile the program then it creates a table having 10 cells and each of them can store an integer value. In the figure see that the index values at the top of each cell starts from 0 and ranges till 9, which specifies the position of each cell in the array. As I said that all the cells are created in successive memory locations. We can access each cell by just specifying the array name and the index number. e.g a[0], a[1] and so on. We can store numbers in each cells by using a "for" loop. See below-
inta[10], i; for(i=0; i<=9; i++){ cout<<"Enter the element :"<<i+1<<":"; cin>>a[i]; }The program segment will store 10 numbers in the array as given below-.
Enter the element : 1: 10
Enter the element : 2: 8
Enter the element : 3: 3
Enter the element : 4: 20
Enter the element : 5: 12
Enter the element : 6: 18
Enter the element : 7: 25
Enter the element : 8: 30
Enter the element : 9: 24
Enter the element : 10: 45
The array will be filled up with the entered numbers 10, 8, 3, 20, 12, 18, 25, 30, 24, and 45. Internally the array would be as below-

See that only changing the index number we mean different variable here as a[0], a[1] and so on. Hence it is possible to use the loop to accept the numbers. In case of individual variable can we do it?
Things to remember:
- While declaring an array the number of cells must be constant e.g int b[10], you can not define as int k=10; then int a[k];
- Array can be initialized while declaring not any other place. e.g int a[]={3, 4, 10, 20}; here see that number of cells kept blank, you can give the number of cells here but better practice not to give it as computer gives it automatically.
Q46.Write a program to store 10 numbers in an array then find the sum of the numbers.
Q47.Write a program to store 10 numbers in an array then display the greatest number.
Q48.Write a program to store 10 numbers in an array then search for a user given number
Q49.Write a program to store 10 numbers in an array then count number of even numbers in it.
Q50.Write a program to store 10 numbers in an array then add 1 with all even numbers and ans subtract 1 from all the odd numbers in it, then display the resulting array.
Program-46
#include <iostream.h> void main(){ int ar[10], i, sum=0; for(i=0; i<= 9; i++){ cout<<"Enter the number:"<<i+1<<":"; cin>>ar[i]; } for(i=0; i<= 9; i++){ sum=sum+ar[i]; } cout<<"The Sum="<<sum; }In this program the array is define as "ar[10]" with 10 cells, the first for loop accepting the numbers from the user into the array. The second for loop reading the content of the array and adding it with "sum". Lastly the sum is displayed. The output of the above program is as below.
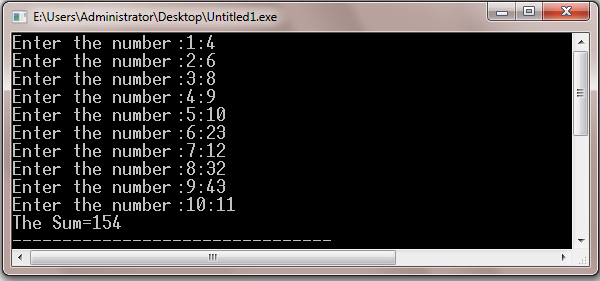
Program-47
#include <iostream.h> void main(){ int ar[10], i, g; for(i=0; i<= 9; i++){ cout<<"Enter the number :"<<i+1<<":"; cin>>ar[i]; } g=ar[0]; for(i=1; i<= 9; i++){ if(ar[i]>g){ g=ar[i]; } } cout<<"The greatest number is ="<<g; }In this program see that the first number is taken a greatest as "g=ar[0]" then in the second loop each number at index number 1 to 9 is comapred with present greatest (g), if it is smaller it is replaced by "ar[i] so it becomes present greatest. At the end of the loop "g" gives the greatest numbers which is displayed.
#include <iostream.h> void main(){ int ar[10], i, k, found=0; for(i=0; i<= 9; i++){ cout<<"Enter the number :"<<i+1<<":"; cin>>ar[i]; } cout<<"Enter the number to be searched :"; cin>>k; for(i=0; i<= 9; i++){ if(ar[i]==k){ found=1; break; } } if(found==1){ cout<<"Number is found"; } else{ cout<<"Number is not found"; } }In this program see the given number "k" is comparing with each number in the array if equal the the variable "found" which was assign to zero at the begining now becomes 1 and exit the loop by the "break" statement. Outside the loop we are checking the value of "found" if it is 1 then the number is found otherwise it is not found.
Program-49
#include <iostream.h> void main(){ int ar[10], i, c=0; for(i=0; i<= 9; i++){ cout<<"Enter the number :"<<i+1<<":"; cin>>ar[i]; } for(i=0; i<= 9; i++){ if(ar[i]%2==0){ c++; } } cout<<"Number of even nos="<<c; }In this program "c" is the accumulator increasing by one when the number is even.
Program-50
#include <iostream.h> void main(){ int ar[10], i; for(i=0; i<= 9; i++){ cout<<"Enter the number :"<<i+1<<":"; cin>>ar[i]; } cout<<"The content of the array:\n" for(i=0; i<= 9; i++){ cout<<ar[i]<<" "; } for(i=0; i<= 9; i++){ if(ar[i]%2==0){ ar[i]=ar[i]=1; } esle{ ar[i]=ar[i]-1; } } cout<<"The content of the array after the operation:\n" for(i=0; i<= 9; i++){ cout<<ar[i]<<" "; } }
In this program the original array is displayed first. Then the required operation is done on the array and the changed content of the array is displayed